Node.js, along with Sequelize ORM, provides a robust environment for developing JavaScript-based applications with database functionality. In this guide, we’ll focus on creating models in Node.js using Sequelize ORM. Models act as the functional database layer, allowing us to connect and interact with database tables efficiently. Each model corresponds to one database table, making Sequelize an essential tool for handling databases in Node.js applications.
Setting Up a Node.js Application
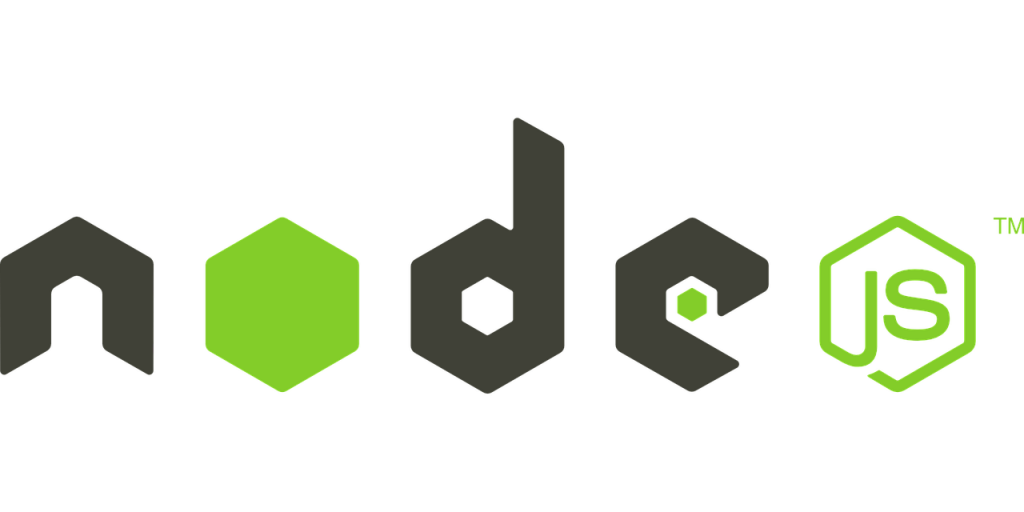
Before we dive into creating models, let’s set up a Node.js application:
- Ensure you have Node.js installed on your system. If not, you can download it from the official Node.js website and follow the installation instructions.
- Create a new folder for your Node.js application. For this example, let’s name it “node-orm.”
- Open your terminal or command prompt and navigate to the “node-orm” folder.
- Create a
package.json
file for your project by running the following command:csharpCopy codenpm init -y
This command generates apackage.json
file with default settings. - Install the necessary packages for your Node.js application. For working with Sequelize, we’ll need to install several packages, including Sequelize itself. Run the following command to install these packages:cssCopy code
npm install sequelize mysql2 express body-parser --save
This command installs Sequelize, the MySQL database driver (mysql2
), and other essential packages for building a web application with Express.js. - With the packages installed, create an
index.js
file inside your “node-orm” folder. This is where we’ll write our application code.
Now, your Node.js application is set up and ready to work with Sequelize ORM.
Connecting to MySQL Database
In our Node.js application, we will connect to a MySQL database. Sequelize supports multiple database systems, but for this example, we’ll use MySQL.
Creating Models in Sequelize ORM
Models can be created in several ways in Sequelize. In the following examples, we’ll explore two methods of defining models.
Method 1: Create a Model in Sequelize ORM
In this method, we use the Sequelize connection variable to create a model.
javascriptCopy code
// Define a User model const User = sequelize.define('User', { id: { type: Sequelize.INTEGER, autoIncrement: true, primaryKey: true, }, name: { type: Sequelize.STRING, }, email: { type: Sequelize.STRING, unique: true, }, rollNo: { type: Sequelize.INTEGER, }, status: { type: Sequelize.BOOLEAN, defaultValue: true, }, created_at: { type: Sequelize.DATE, defaultValue: Sequelize.NOW, }, updated_at: { type: Sequelize.DATE, defaultValue: Sequelize.NOW, }, });
In the above code, we define a User
model with various fields like id
, name
, email
, rollNo
, status
, created_at
, and updated_at
. Sequelize will create a corresponding table in the connected database named “tbl_users” with these columns.
If you don’t want timestamp columns like created_at
and updated_at
, you can set them to false
in the configuration object.
Method 2: Using Class-Based Concept of Sequelize
You can also create models using Sequelize’s class-based approach. Here’s an example:
javascriptCopy code
const { DataTypes, Model } = require('sequelize'); class User extends Model {} User.init( { id: { type: DataTypes.INTEGER, autoIncrement: true, primaryKey: true, }, name: { type: DataTypes.STRING, }, email: { type: DataTypes.STRING, unique: true, }, }, { sequelize, modelName: 'User', tableName: 'tbl_users', // Specify the table name if different from the model name timestamps: false, // Disable timestamp columns } );
This code demonstrates creating a User
model using Sequelize’s class-based approach, allowing for more extensive customization.
Complete Source Code – Model in Node Js ORM
Certainly, here’s the complete source code for creating a model in Node.js using Sequelize ORM:
javascriptCopy code
// Import required packages and dependencies const Sequelize = require('sequelize'); // Create a Sequelize instance and connect to the MySQL database const sequelize = new Sequelize('your_database_name', 'your_username', 'your_password', { host: 'localhost', dialect: 'mysql', }); // Define a User model const User = sequelize.define('User', { id: { type: Sequelize.INTEGER, autoIncrement: true, primaryKey: true, }, name: { type: Sequelize.STRING, }, email: { type: Sequelize.STRING, unique: true, }, rollNo: { type: Sequelize.INTEGER, }, status: { type: Sequelize.BOOLEAN, defaultValue: true, }, created_at: { type: Sequelize.DATE, defaultValue: Sequelize.NOW, }, updated_at: { type: Sequelize.DATE, defaultValue: Sequelize.NOW, }, }); // Synchronize the model with the database sequelize.sync() .then(() => { console.log('Model synchronized with the database.'); }) .catch((err) => { console.error('Error syncing model with the database:', err); });
In this code:
- We import the Sequelize package.
- We create a Sequelize instance and configure it to connect to your MySQL database. Replace
'your_database_name'
,'your_username'
, and'your_password'
with your actual database credentials. - We define a
User
model with various fields such asid
,name
,email
,rollNo
,status
,created_at
, andupdated_at
. - We synchronize the model with the database using
sequelize.sync()
. This ensures that the model’s structure matches the database schema.
Make sure to replace the placeholder values with your actual database information. This code creates a User
model that corresponds to a “tbl_users” table in your MySQL database.
Back to a terminal and start server using nodemon.
To start your server using nodemon
, follow these steps in your terminal:
- Make sure you are in the root directory of your Node.js application, where your
index.js
file is located. - If you haven’t already installed
nodemon
globally on your system, you can do so with the following command:Copy codenpm install -g nodemon
This installsnodemon
as a global package, making it available to all your Node.js projects. - Once
nodemon
is installed, you can start your Node.js server by running the following command:Copy codenodemon index.js
Replaceindex.js
with the name of your main application file if it’s named differently. - After running the command,
nodemon
will start monitoring your files for changes. If you make any changes to your code and save the files,nodemon
will automatically restart your server to reflect the updated code.
You should see output in your terminal indicating that your server is running, and any errors or logs generated by your application will also be displayed there.
Now, your Node.js server should be up and running, and you can access it by navigating to the specified address in your web browser or using API testing tools if you’re building an API.
Perform Database Operations with Models
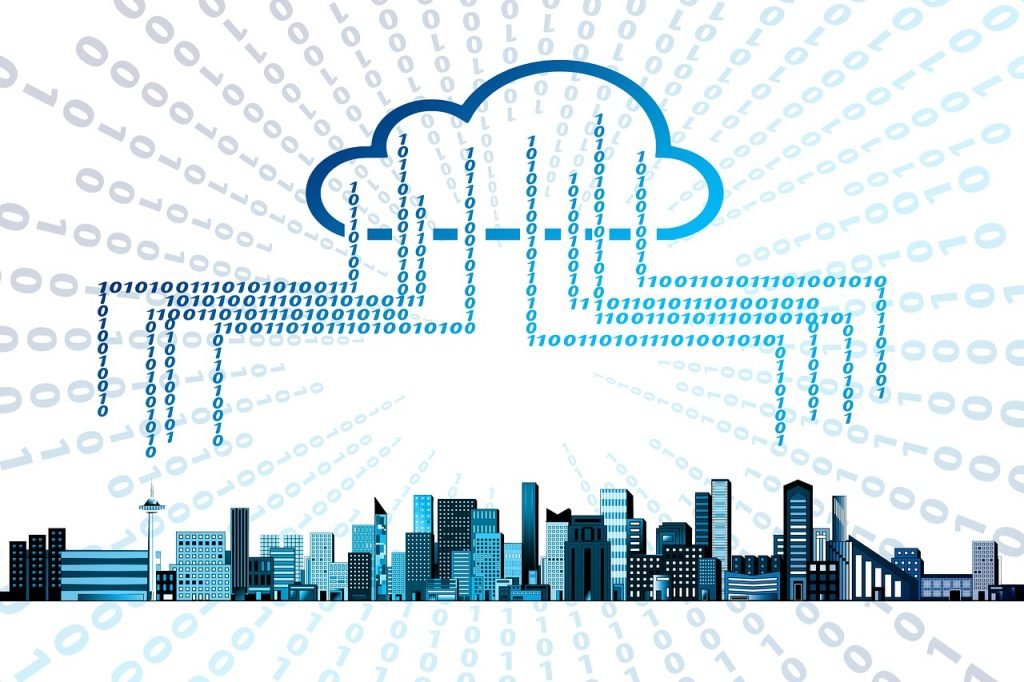
Performing database operations with models in Sequelize is a fundamental aspect of building Node.js applications. Below, we’ll cover common database operations like data insertion, retrieval (selection), updating, and deletion using Sequelize models.
Assuming you have already created a Sequelize model named User
as demonstrated earlier, we can proceed with these operations:
Data Insertion
To insert data into the database using your Sequelize model, you can follow these steps:
javascriptCopy code
// Create a new User instance with data const newUser = User.build({ name: 'John Doe', email: 'john@example.com', rollNo: 12345, status: true, }); // Save the new user to the database newUser.save() .then((savedUser) => { console.log('User saved to the database:', savedUser.toJSON()); }) .catch((error) => { console.error('Error saving user:', error); });
In this code, we create a new User
instance with the desired data and then call the save()
method to insert it into the database.
Data Retrieval (Selection)
To retrieve data from the database using your Sequelize model, you can use methods like findOne()
or findAll()
. Here’s an example of retrieving a single user by their email:
javascriptCopy code
User.findOne({ where: { email: 'john@example.com' } }) .then((user) => { if (user) { console.log('Found user:', user.toJSON()); } else { console.log('User not found.'); } }) .catch((error) => { console.error('Error retrieving user:', error); });
This code uses the findOne()
method to retrieve a user with the specified email address.
Data Updating
To update data in the database using your Sequelize model, you can follow these steps:
javascriptCopy code
// Find a user to update by their email User.findOne({ where: { email: 'john@example.com' } }) .then((user) => { if (user) { // Update the user's name user.name = 'Updated Name'; return user.save(); } else { console.log('User not found.'); } }) .then((updatedUser) => { if (updatedUser) { console.log('User updated successfully:', updatedUser.toJSON()); } }) .catch((error) => { console.error('Error updating user:', error); });
In this code, we first find the user we want to update, make the necessary changes, and then call save()
to save the updates to the database.
Data Deletion
To delete data from the database using your Sequelize model, you can use the destroy()
method:
javascriptCopy code
// Find a user to delete by their email User.findOne({ where: { email: 'john@example.com' } }) .then((user) => { if (user) { return user.destroy(); } else { console.log('User not found.'); } }) .then(() => { console.log('User deleted successfully.'); }) .catch((error) => { console.error('Error deleting user:', error); });
In this code, we first find the user to delete and then call the destroy()
method on that user instance.
These are the basic database operations you can perform with Sequelize models. Depending on your application’s requirements, you can build more complex queries and operations using Sequelize’s powerful querying capabilities.
Conclusion
In this guide, we’ve covered setting up a Node.js application, connecting it to a MySQL database, and creating models in Sequelize ORM. Sequelize provides a powerful toolset for working with databases in Node.js, making it easier to manage and interact with your data. With these foundations in place, you can now perform various database operations, such as data insertion, selection, updates, and deletions, using Sequelize models in your Node.js application.